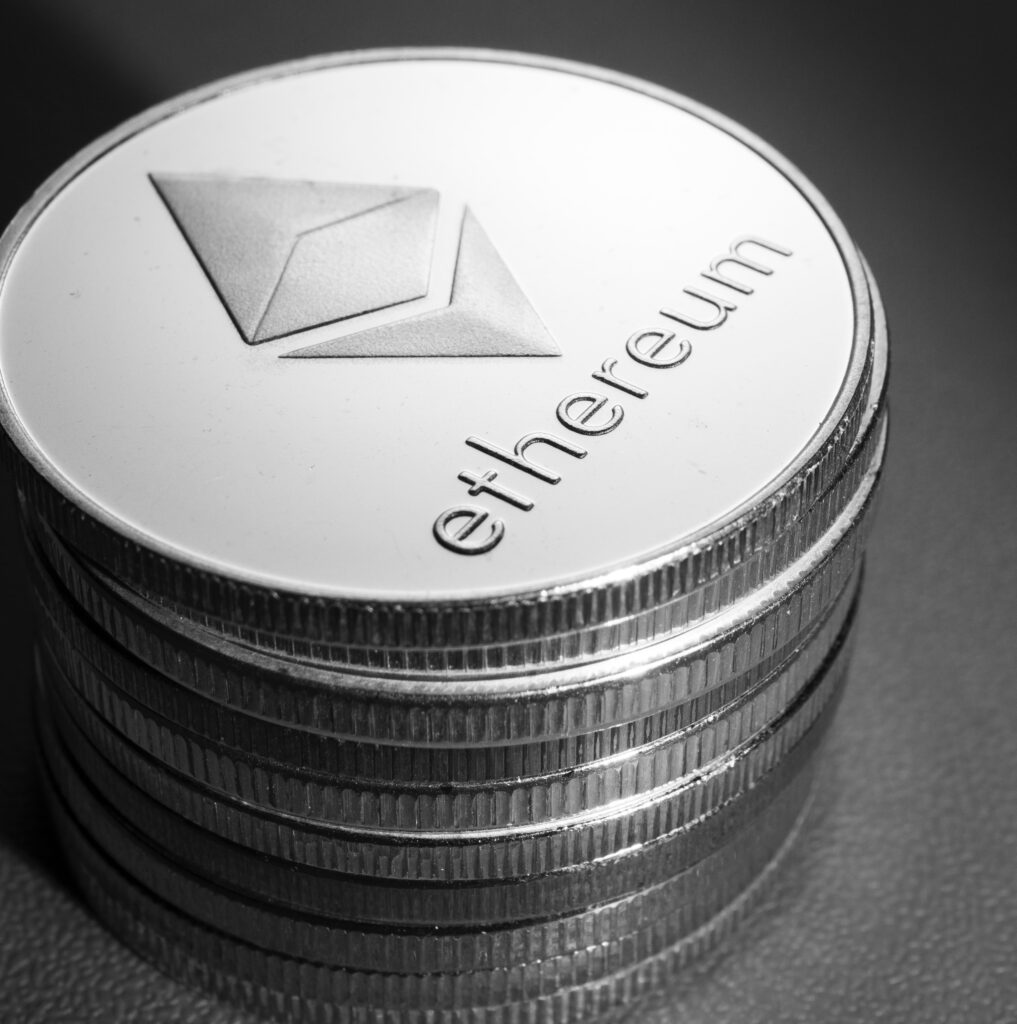
Web3 is a term that refers to the next generation of the World Wide Web, where decentralized technologies are used to build applications that are more open, transparent, and secure. Web3 incorporates blockchain technology and smart contracts to enable peer-to-peer transactions, decentralized identity, and decentralized applications (dApps). In the context of C# and .NET development, Nethereum is a powerful library that allows you to interact with the Ethereum blockchain using C#.
Started with QuickNode
QuickNode is a platform that provides infrastructure and hosting services for blockchain and Web3 applications. It allows developers to easily deploy and manage their own nodes, which are essential for interacting with various blockchain networks. QuickNode supports a wide range of blockchain networks, including Ethereum, Bitcoin, Binance Smart Chain, and more.
By using QuickNode, developers can access the full functionality of blockchain networks without the need to set up and maintain their own infrastructure. QuickNode offers reliable and scalable node hosting, real-time monitoring, API access, and developer tools to simplify the process of building decentralized applications (dApps) and interacting with blockchain networks.
Step1: Go to https://QuickNode.com/, create account and verify your email.
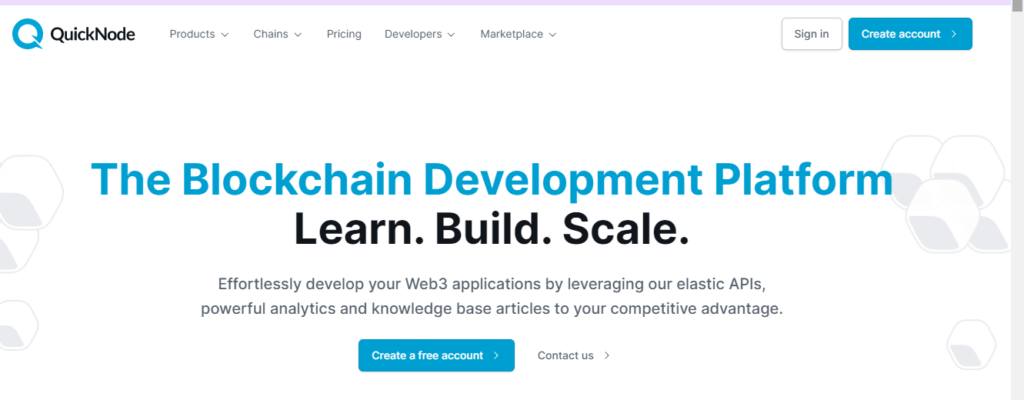
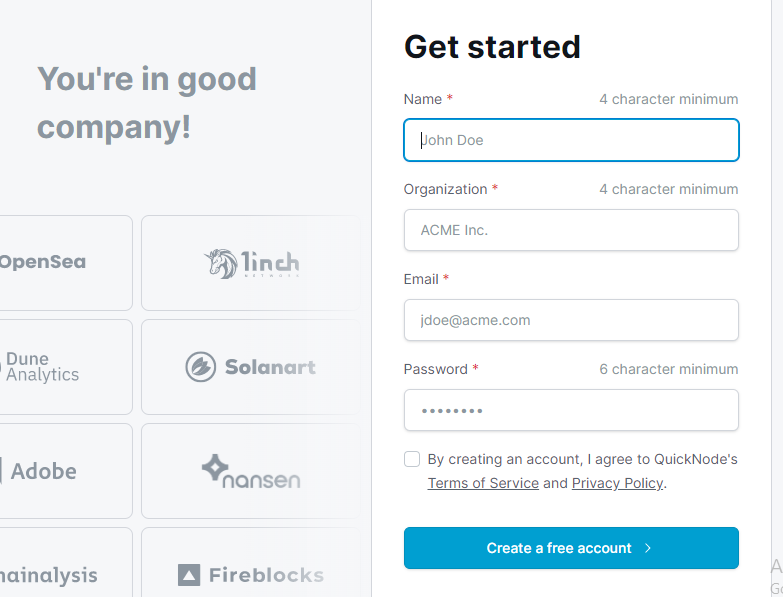
Step2: Select your Plan
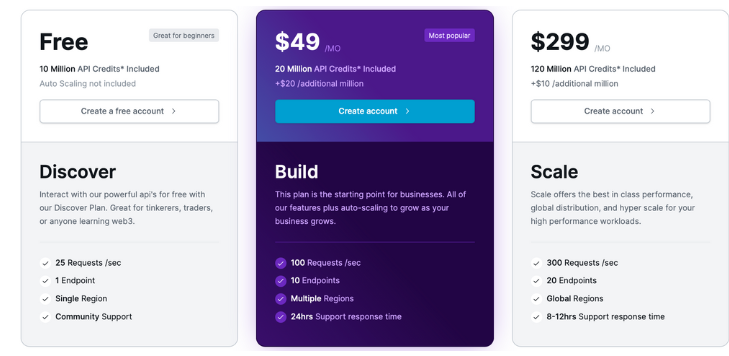
Step3: Create your endpoint
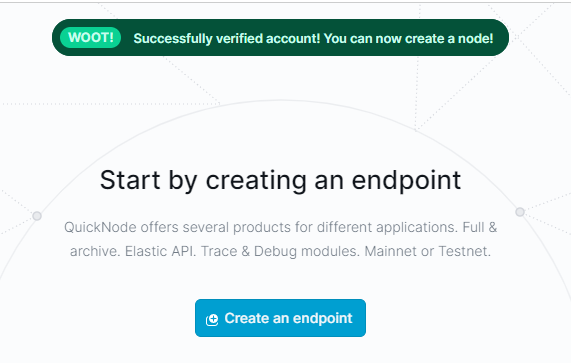
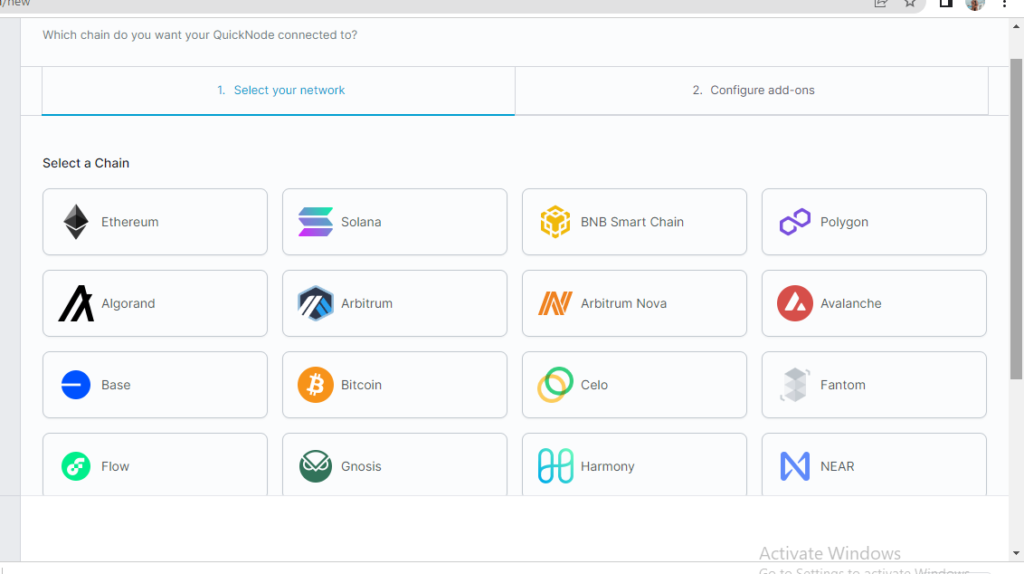
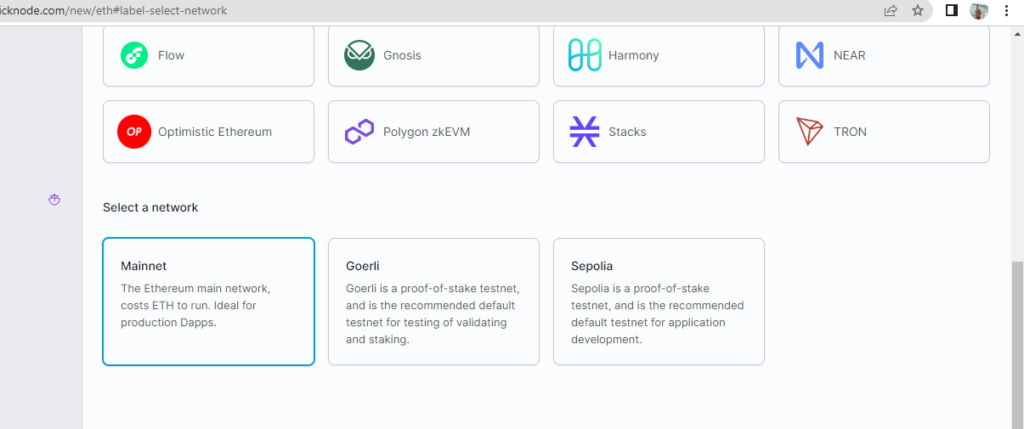
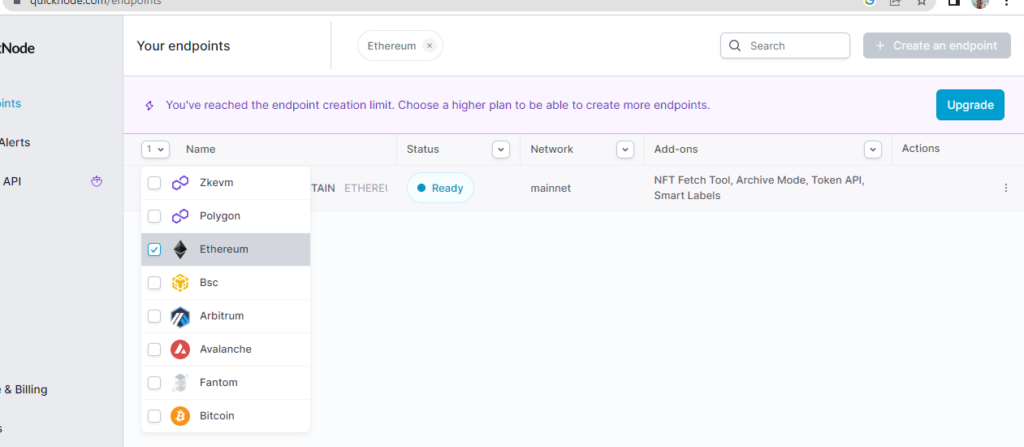
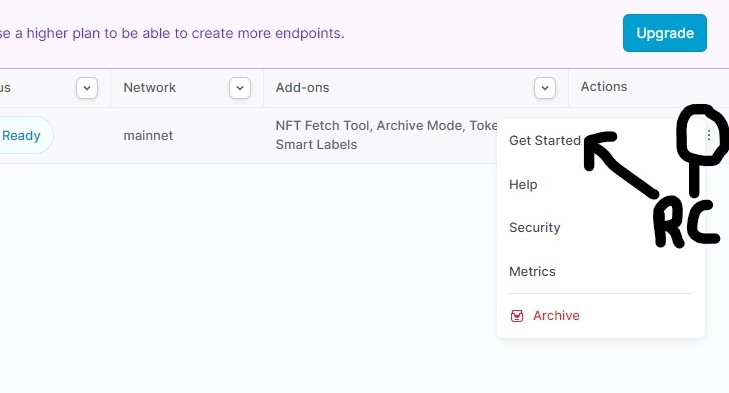
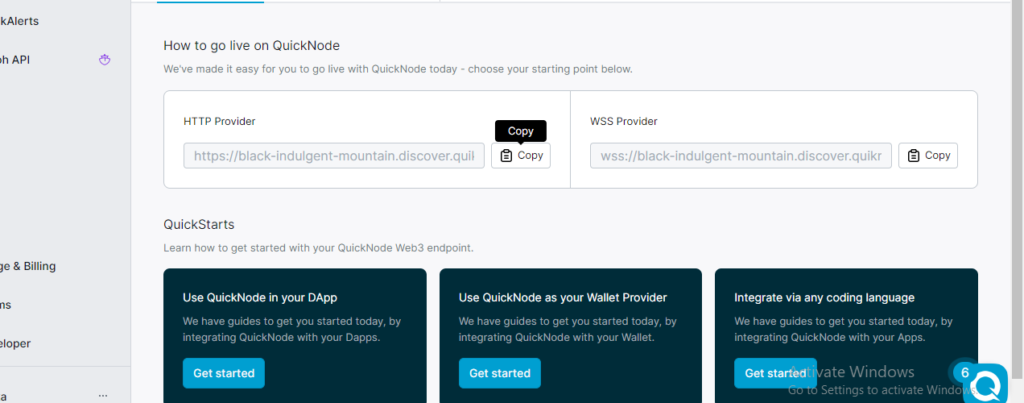
, the URL in the HTTP provider “https://black-indulgent-mountain.discover.quiknode.pro/d0129a8c1559a7d8f6c69a6641591f0a7813e561/” is passed as a parameter when creating a new instance of the Web3 class. This URL specifies the endpoint of the QuikNode service that hosts the Ethereum node.
How to connect to Ethereum using .NET (Nethereum)
Nethereum is a .NET integration library for Ethereum, which allows developers to interact with the Ethereum blockchain using .NET and C#. It provides a comprehensive set of tools and functionalities for building decentralized applications (dApps) and interacting with Ethereum smart contracts.
Installing Nethereum
Nethereum requires .NET Core or .NET Framework(4.5.1+) installed. We’ll install .NET Core SDK to make Nethereum work. Download and install the .NET Core SDK ver 3.1 based on your operating system. Then go to your terminal/command line and type the following.
$ dotnet new console -o nethereuma
This will create a new .NET application in the current directory. You can give your application any name
Now cd into your application.
$ cd nethereuma
Add package reference to Nethereum.Web3
$ dotnet add package Nethereum.Web3
This might take a while. After the package reference is added, download/update the package by typing the following.
$ dotnet restore
If everything goes right, Nethereum will be added to your system with nethereum web3 package.
Booting our Ethereum node
After you’ve created your free ethereum endpoint, copy your HTTP Provider endpoint:
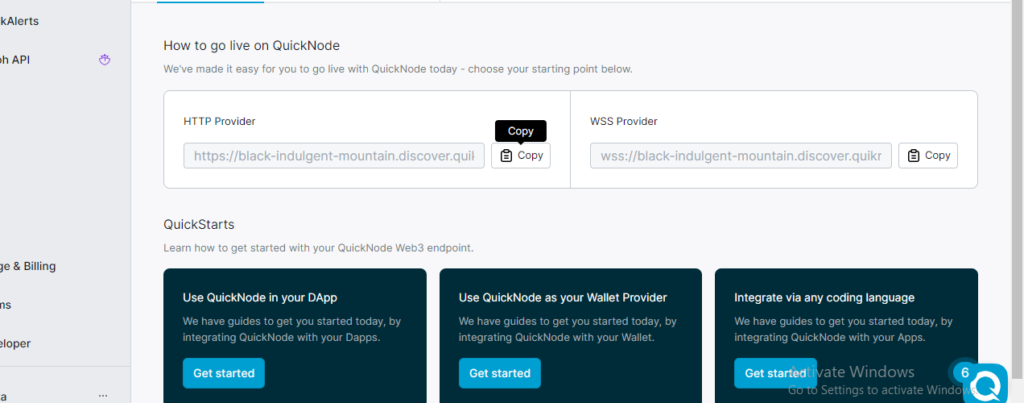
You’ll need this later, so copy it and save it.
Connecting with Ethereum
Now go to your .NET app folder and open the Program.cs C# file in a text editor .
Connect with Ethereum and then get the latest block number from the Ethereum blockchain.
class Program
{
static void Main()
{
GetBlockNumber().Wait();
}
static async Task GetBlockNumber()
{
var web3 = new Web3("ADD_YOUR_ETHEREUM_NODE_URL");
var latestBlockNumber = await web3.Eth.Blocks.GetBlockNumber.SendRequestAsync();
Console.WriteLine($"Latest Block Number is: {latestBlockNumber}");
}
}
Generate a Ethereum Address
While signing in to any platform on the internet, you need to authenticate using a combination of credentials. Consider an Ethereum address as your username and a corresponding private key as the password. While your Ethereum address is public and can be shared, the private key must always be kept secret. Using this combination lets you interact with the Ethereum blockchain. An Ethereum address is your identity on the blockchain, and it looks like this “0x6E0d01A76C3Cf4288372a29124A26D4353EE51BE”.
static void GenerateEthereumAddress()
{
// Generate a new Ethereum account
var ecKey = Nethereum.Signer.EthECKey.GenerateKey();
var privateKey = ecKey.GetPrivateKey();
var address = ecKey.GetPublicAddress();
// Output the generated address and private key
Console.WriteLine("Ethereum Address: " + address);
Console.WriteLine("Private Key: " + privateKey);
}
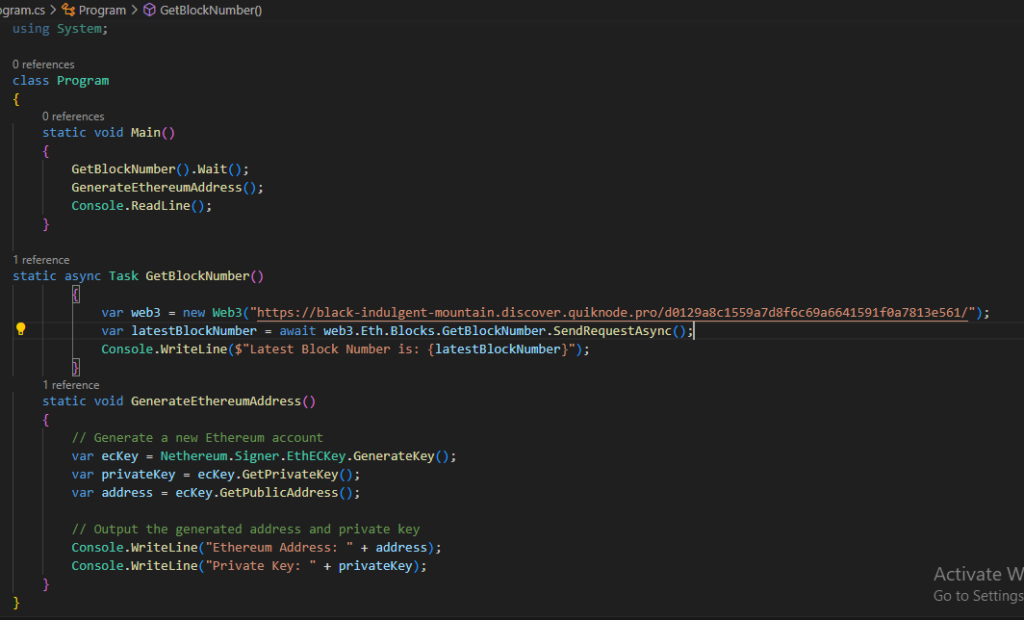
Run the code
