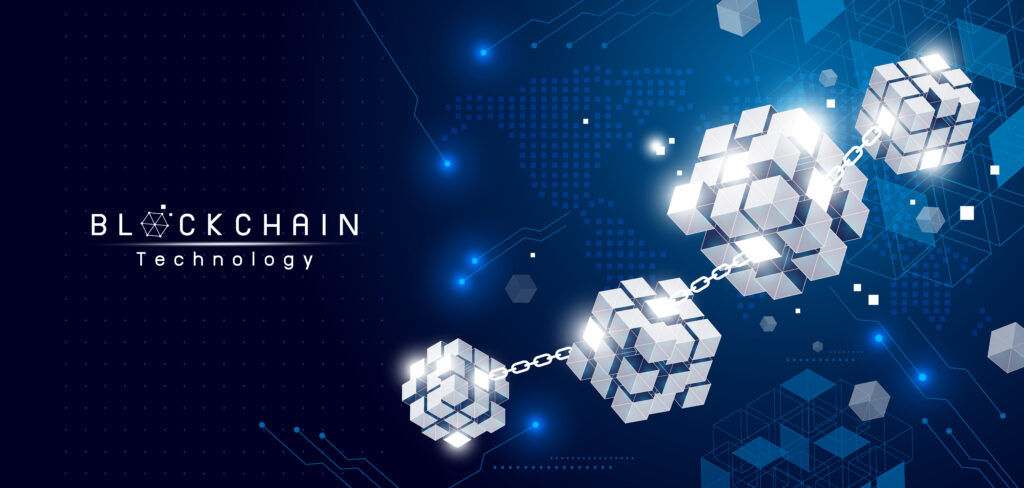
If we want to understand the Blockchain we can say that in simple terms, a blockchain is a digital ledger of transactions that is decentralized, meaning that it is not controlled by a central authority, like a bank or government. Instead, it is maintained by a network of computers that all work together to verify and record transactions.
To understand how a blockchain works, imagine a group of people who are all keeping track of their transactions on a piece of paper. Each time someone makes a transaction, they write it down on their paper, along with the time, amount, and other details. This creates a record of all the transactions that have taken place.
Now imagine that instead of just one piece of paper, there are thousands of them, each being kept by a different person. Each time a transaction is made, it is recorded on all of the pieces of paper, and each person checks to make sure that the transaction is valid. If everything checks out, the transaction is added to the ledger, and everyone has a copy of the updated ledger.
This is essentially how a blockchain works, except instead of paper, it uses digital blocks of information that are linked together in a chain. Each block contains a record of several transactions, and when a new block is added to the chain, it is verified by the network of computers to make sure that it is valid. Once it has been verified, it is added to the chain and cannot be changed or deleted.
One of the key features of blockchain technology is its security. Because the ledger is decentralized and maintained by a network of computers, it is extremely difficult for anyone to hack or alter the information. This makes it a great way to store sensitive information, like financial transactions or personal data.
Another advantage of blockchain is its transparency. Because every transaction is recorded and verified by the network, it is possible to trace the history of a particular asset or transaction all the way back to its origin. This can be particularly useful in industries like supply chain management, where it is important to track the movement of goods from one place to another. Blockchain is a digital ledger of transactions that is decentralized and maintained by a network of computers. It is secure, transparent, and can be used for a wide range of applications. While it may seem complex at first, the basic idea is relatively simple, and it has the potential to revolutionize the way we store and share information.
So above we tried to understand what is blockchain and how its used in a layman’s way. Now we will understand it from the prespective of technical view.
a blockchain is a decentralized ledger of transactions that are secured through cryptographic protocols. In other words, it’s a digital database that is distributed across a network of computers, with each computer storing a copy of the database.
The key feature of a blockchain is its decentralized nature. Traditional databases are centralized, meaning that they are owned and operated by a single entity, such as a bank or government. This makes them vulnerable to hacking, censorship, and corruption. In contrast, a blockchain is decentralized, which means that no single entity has control over the data. Instead, it is controlled by the network of computers that participate in the system.
One of the most popular implementations of blockchain technology is Bitcoin. Bitcoin is a decentralized digital currency that operates on a blockchain. It was created in 2009 by an unknown person using the pseudonym Satoshi Nakamoto. Since then, it has grown in popularity, with a market capitalization of over $1 trillion at the time of writing.
The Bitcoin blockchain is a distributed ledger that records every Bitcoin transaction. Each transaction is verified by a network of computers, known as nodes, that are connected to the blockchain. Nodes work together to validate transactions by solving complex mathematical equations, a process known as mining. When a block of transactions is validated, it is added to the blockchain, creating a permanent and unalterable record.
The security of a blockchain is ensured by a combination of cryptographic protocols, including hash functions and digital signatures. Hash functions are algorithms that take an input and generate a fixed-size output, known as a hash. Each block in the blockchain contains a hash of the previous block, creating a chain of blocks that are linked together. This creates an immutable record that cannot be tampered with.
Digital signatures are used to ensure that transactions are authentic. Each user on the network has a public key and a private key. The public key is used to verify the digital signature, while the private key is used to create the signature. When a user makes a transaction, they use their private key to create a digital signature that is attached to the transaction. This ensures that the transaction is authentic and cannot be forged.
Another key feature of a blockchain is its transparency. Because the ledger is distributed across a network of computers, anyone can view the transactions on the blockchain. This makes it ideal for use cases where transparency is important, such as supply chain management or voting systems.
Blockchain technology has the potential to revolutionize many industries, including finance, healthcare, and logistics. Its decentralized and secure nature makes it ideal for storing sensitive information, such as medical records or financial transactions. However, it’s important to note that blockchain technology is still in its early stages and there are many challenges that need to be overcome, such as scalability and energy consumption.
Let’s make a simple Blockchain program in Steps.
Step 1: Create the Block class
The first step is to create a Block class that will store the data for each block in the blockchain. Here’s an example:
public class Block
{
public int Index { get; set; }
public DateTime Timestamp { get; set; }
public string Data { get; set; }
public string PreviousHash { get; set; }
public string Hash { get; set; }
}
Each block will have an index, a timestamp, some data, a hash of the previous block in the chain, and a hash of the current block.
Step 2: Create the Blockchain class
Next, we’ll create a Blockchain class that will manage the chain of blocks. Here’s an example:
public class Blockchain
{
private List<Block> _chain;
public Blockchain()
{
_chain = new List<Block>();
AddGenesisBlock();
}
private void AddGenesisBlock()
{
_chain.Add(new Block
{
Index = 0,
Timestamp = DateTime.Now,
Data = "Genesis Block",
PreviousHash = null,
Hash = CalculateHash(0, DateTime.Now, "Genesis Block", null)
});
}
private string CalculateHash(int index, DateTime timestamp, string data, string previousHash)
{
using (SHA256 sha256 = SHA256.Create())
{
string input = index + timestamp.ToString("yyyy-MM-dd HH:mm:ss.fff") + data + previousHash;
byte[] bytes = sha256.ComputeHash(Encoding.UTF8.GetBytes(input));
StringBuilder builder = new StringBuilder();
for (int i = 0; i < bytes.Length; i++)
{
builder.Append(bytes[i].ToString("x2"));
}
return builder.ToString();
}
}
}
The Blockchain class contains a list of blocks, with the first block being the genesis block. We also have a private method, CalculateHash, that takes in the index, timestamp, data, and previous hash and returns the SHA256 hash of that input.
Step 3: Add blocks to the chain
Now that we have our classes set up, we can add some blocks to the chain. Here’s an example:
Blockchain blockchain = new Blockchain();
blockchain.AddBlock("Transaction 1");
blockchain.AddBlock("Transaction 2");
The AddBlock method takes in some data, creates a new block with that data, and adds it to the chain.
Step 4: Verify the blockchain
Finally, we can verify that our blockchain is valid by checking that each block’s hash matches the previous block’s hash. Here’s an example:
bool isValid = true;
for (int i = 1; i < blockchain.Count; i++)
{
Block currentBlock = blockchain[i];
Block previousBlock = blockchain[i - 1];
if (currentBlock.PreviousHash != previousBlock.Hash)
{
isValid = false;
break;
}
}
Console.WriteLine($"Is blockchain valid? {isValid}");
This code loops through each block in the chain and checks that its PreviousHash matches the previous block’s Hash. If any of the blocks are invalid, the isValid flag is set to false.
And that’s it! With these simple steps, we’ve created a functioning blockchain in C#.
In conclusion, a blockchain is a decentralized ledger of transactions that is secured through cryptographic protocols. It uses a network of computers to validate transactions and ensure the security of the ledger. Its transparency and security make it ideal for a wide range of applications, but there are still many challenges that need to be addressed before it can reach its full potential.