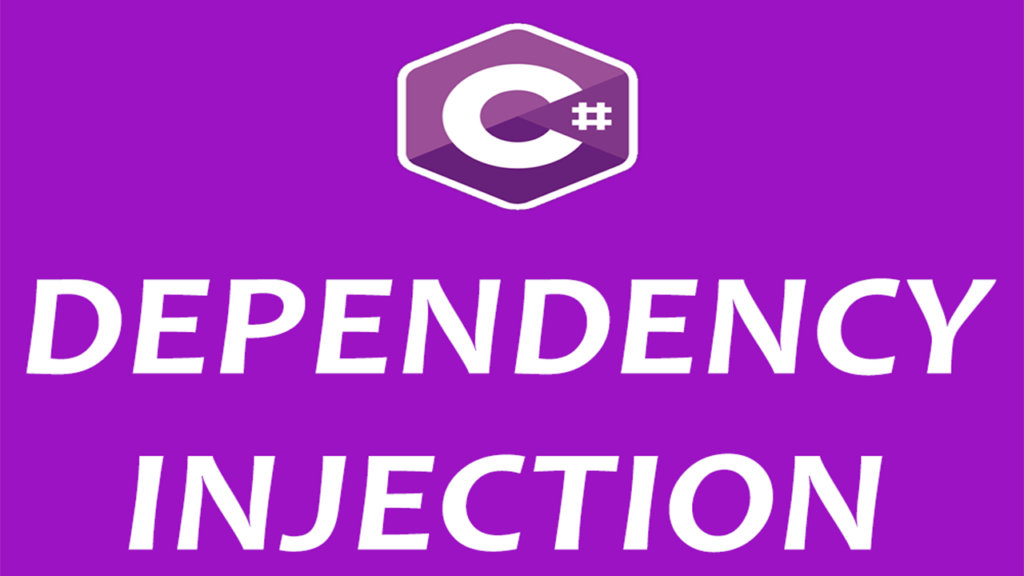
Introduction
As software systems grow in complexity, managing dependencies becomes increasingly challenging. One solution to this problem is Dependency Injection (DI). DI is a technique that helps to decouple components in a system by removing the responsibility of creating and managing dependencies from the components themselves.
In this article, we’ll take a closer look at DI in C#. We’ll define what DI is, its features, and why it’s beneficial. Finally, we’ll provide an example of how to implement DI in a C# application.
What is Dependency Injection?
Dependency Injection (DI) is a design pattern in object-oriented programming that aims to make the code more modular and maintainable by reducing the coupling between components. In C#, DI is achieved by using a third-party DI container library or by manually injecting dependencies into classes.
Dependency Injection involves three main components:
- Dependency: A dependency is an object that is needed by another object to perform its tasks.
- Injection: Injection is the process of passing a dependency to an object.
- Container: A container is responsible for managing the dependencies and their lifecycles.
By using DI, the application becomes more modular, testable, and maintainable.
Features of Dependency Injection
There are several features of DI that make it useful in software development:
Decouples components
DI decouples components in a system by removing the responsibility of creating and managing dependencies from the components themselves. This enables components to be more modular and flexible, allowing for easier testing and maintenance.
Increases code reuse
DI increases code reuse by promoting the use of smaller, more focused classes with clear responsibilities. This enables the reuse of code across multiple components in the application.
Facilitates testing
DI facilitates testing by allowing dependencies to be mocked or replaced with test implementations. This makes it easier to test individual components in isolation without relying on the behavior of other components.
Enables configuration flexibility
DI enables configuration flexibility by allowing dependencies to be swapped out at runtime. This makes it easier to change the behavior of the application without modifying its source code.
Let’s take a look at an example of how to use DI in a C# application. Suppose we have a UserService
class that needs to send emails to users. Instead of creating an EmailService
instance inside the UserService
, we can use DI to inject the EmailService
as a dependency into the UserService
.
First, we’ll define an interface for the EmailService
:
public interface IEmailService { void SendEmail(string to, string subject, string body); }
Next, we’ll define the EmailService
class that implements this interface:
public class EmailService : IEmailService
{
public void SendEmail(string to, string subject, string body)
{
// Implementation to send email
}
}
Now, we’ll modify the UserService
to accept an IEmailService
instance as a constructor parameter:
public class UserService
{
private readonly IEmailService _emailService;
public UserService(IEmailService emailService)
{
_emailService = emailService;
}
Conclusion:
In conclusion, Dependency Injection (DI) is a technique used in software development to manage dependencies between objects and components. DI enables decoupling of components, increased code reuse, easier testing, and configuration flexibility. By separating the creation of an object from its use, DI makes code more modular, flexible, and easier to test and maintain.