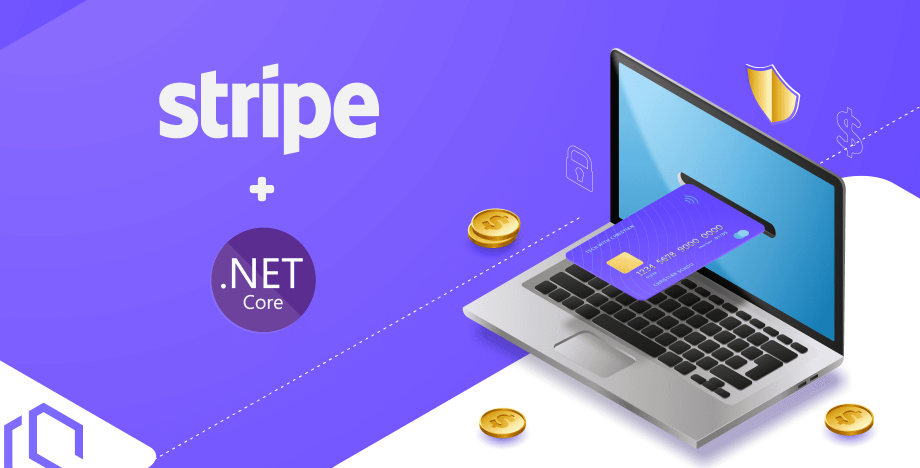
Stripe is a popular payment gateway that makes it easy to accept credit card payments.Stripe enables businesses to accept online payments, process subscriptions, and manage their finances. It provides a secure and easy-to-use platform for processing credit card transactions, bank transfers, and other forms of online payments. Stripe offers a range of features and integrations, including support for over 135 currencies and a variety of payment methods. Stripe also provides robust APIs and developer tools that make it easy to customize payment flows and integrate with other systems. With Stripe, businesses of all sizes can accept payments online and grow their business.
Following are the steps to integrate Stripe into your .NET 6 C# application
In order to move on and accept Stripe payments in .NET, we have to get some API keys to authenticate with. This is possible when you got a Stripe account.
STEP 1 : Signup for/Create a new Stripe account
If you do not already have an account, you can register for one here. https://dashboard.stripe.com/register
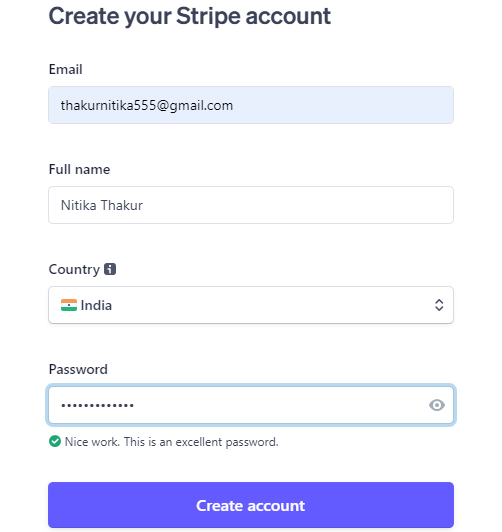
Fill details and click on register .After register this type of dashboard will appear.
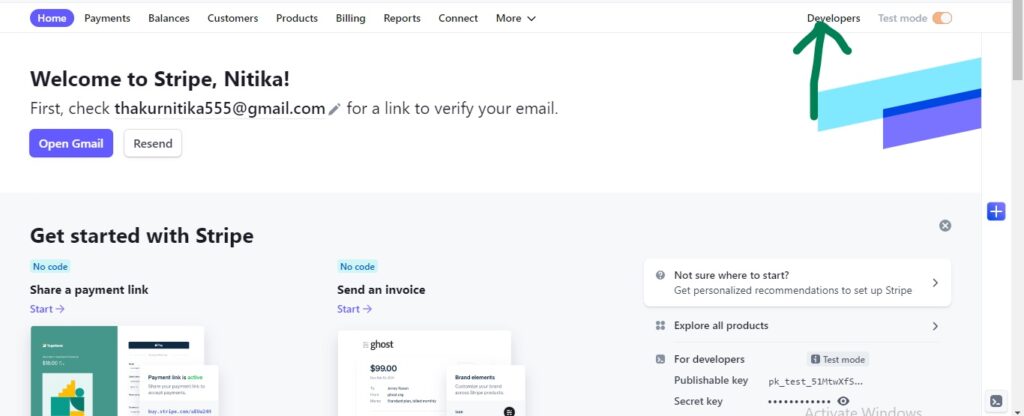
Once you’ve created your account, go to the Developers section and navigate to the API keys page to get the stripe API keys: Publishable Key and Secret Key.
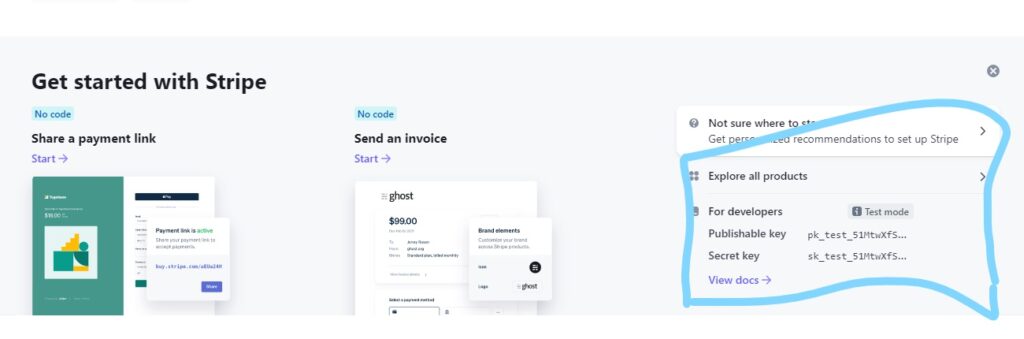
What are the keys used for?
- The
Publishable key
is used by the client application. This could be a web or mobile app making connections. - The
Secret key
is used in the authentication process at the backend server (API Server). By default, we use this key to perform any of the requests between our Web API and Stripe.
STEP 2 : Install the Stripe.NET library using NuGet package manager In Visual Studio, right-click on your project in the Solution Explorer and select Manage NuGet Packages. In the NuGet Package Manager window, search for Stripe.NET and install the latest version.
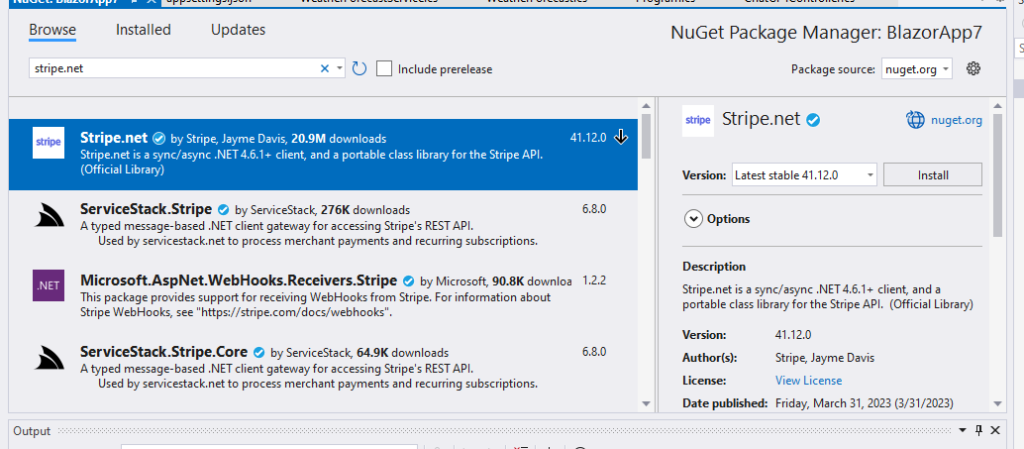
STEP 3: Add the necessary configuration settings in the appsettings.json file .In your appsettings.json file, add the following configuration settings:
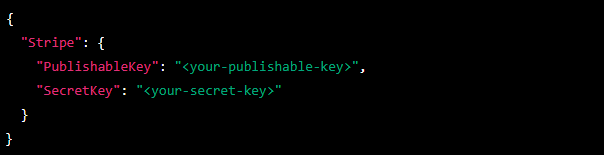
Replace <your-publishable-key>
and <your-secret-key>
with the values you obtained from the Stripe API keys page.
RegisterServices
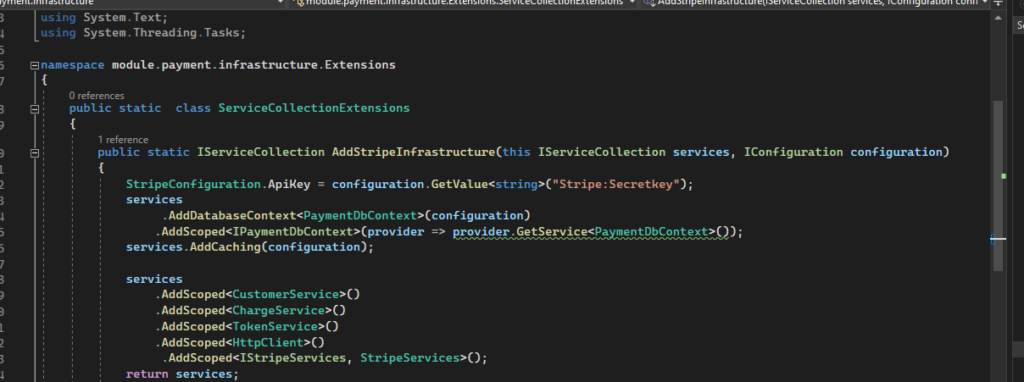
Now create Checkout form in blazor for entering CustomerDetails
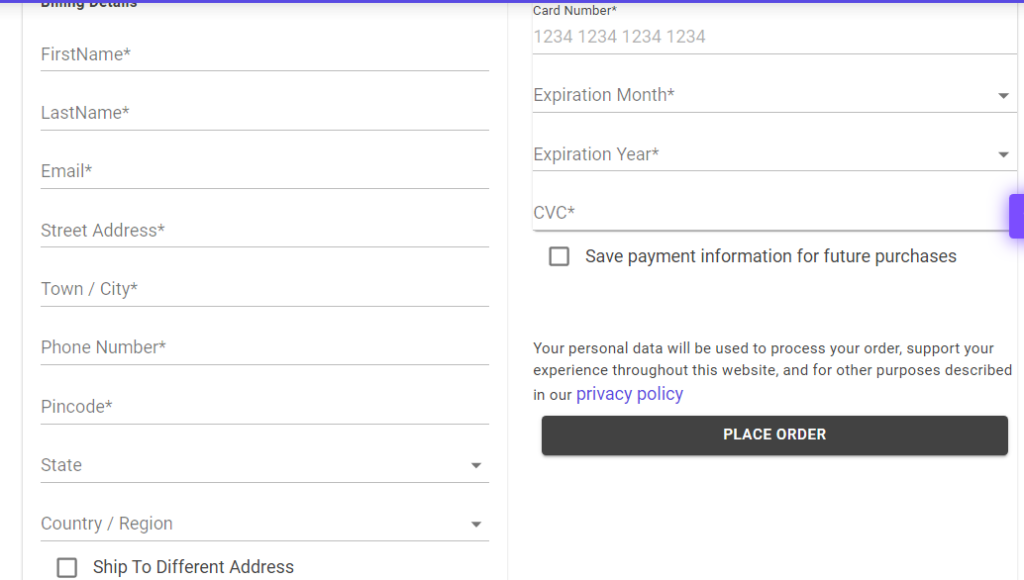
when we click on PlaceOrder button Checkout method will call .On the server side we call Stripe Services .
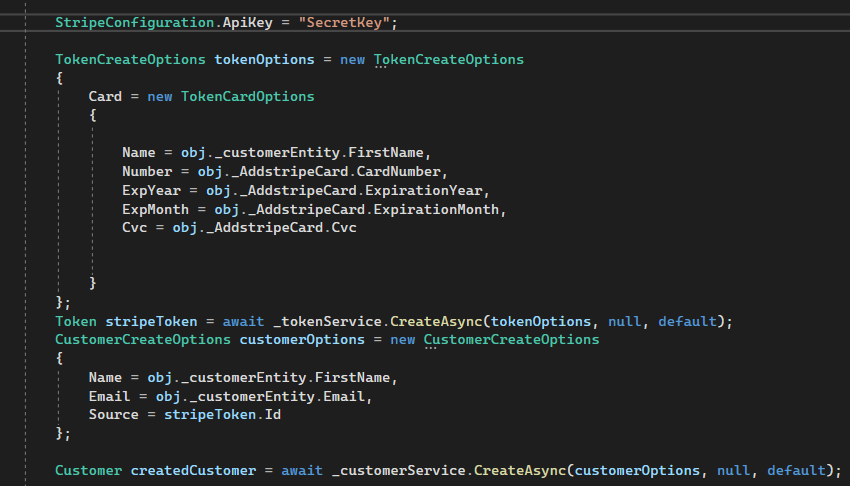
First, create an object of credit card to generate token. While creating customer object we need this token.Set StripeTokenID to the customer object. This code will add customer on Stripe.
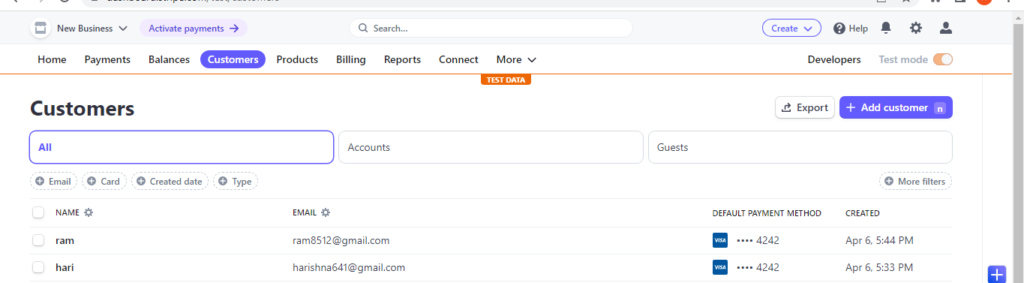
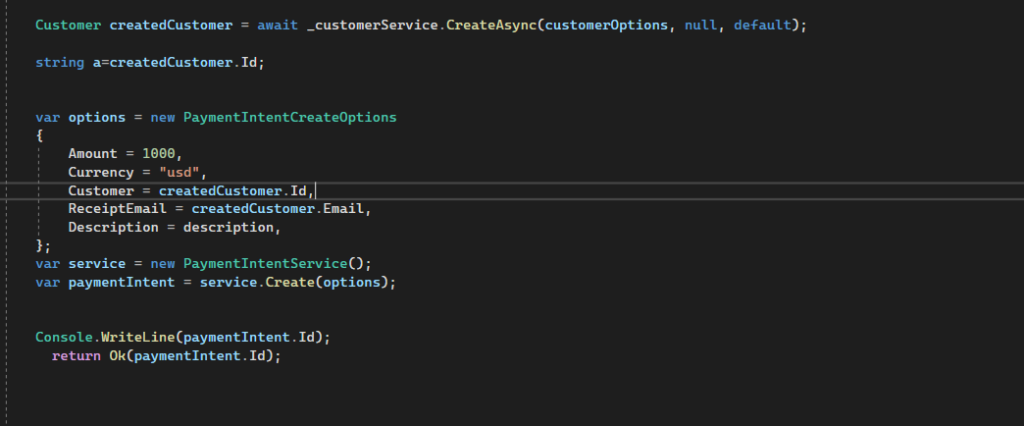
PaymentIntentCreateOptions
is a class in the Stripe API that contains various options for creating a new Payment Intent.
In Stripe, a Payment Intent represents the process of collecting a payment from a customer. When creating a Payment Intent, you can specify various options such as the amount to charge, the currency to use, the payment method to attach to the intent, and other details.
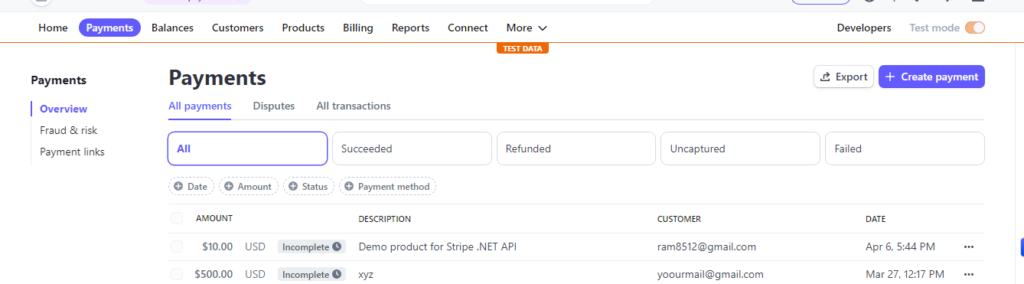