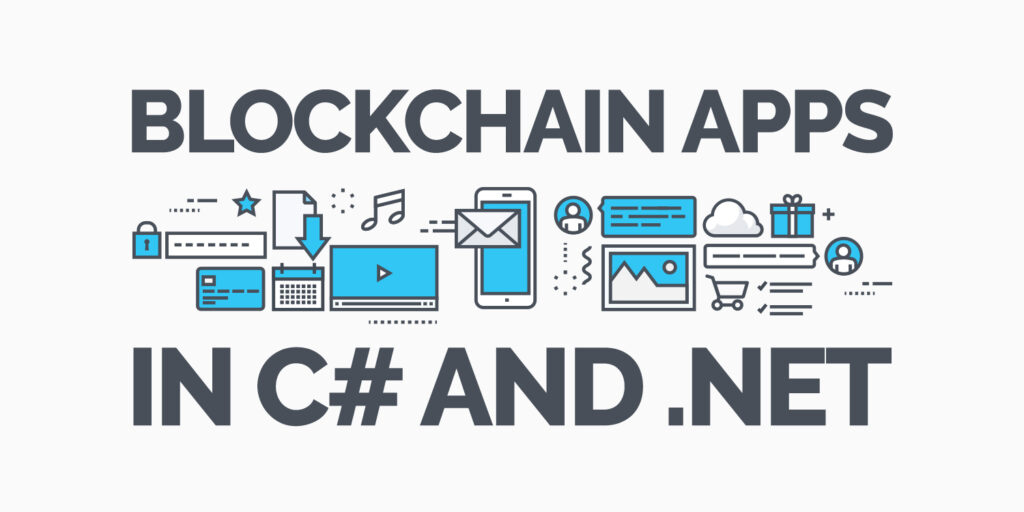
Blockchain technology has revolutionized numerous industries by providing a secure, decentralized, and transparent platform for conducting transactions and maintaining records. With the increasing demand for blockchain applications, integrating blockchain into web applications has become essential for many businesses. In this article, we will explore how to implement blockchain in ASP.NET Core, one of the most popular web development frameworks, using the C# programming language. We will cover the key concepts of blockchain and walk through code examples to demonstrate its implementation.
Blockchain is a distributed ledger technology that enables the creation of a decentralized, tamper-proof, and transparent system for recording transactions. It consists of a chain of blocks, where each block contains a set of transactions and a reference to the previous block. The key features of blockchain include immutability, transparency, and consensus.
we will use Nethereum, Nethereum is a popular Ethereum library for .NET
Now for the Part of Implementing the Blockchain Logic.
First we will create a new folder called “Blockchain” in the project root directory.
Now inside the blockchain folder we will add new class file Block.cs This class represents a block in the blockchain.
public class Block
{
public int Index { get; set; }
public DateTime Timestamp { get; set; }
public string Data { get; set; }
public string PreviousHash { get; set; }
public string Hash { get; set; }
}
Below is the description of the Class Block Properties.
Index: It is an integer property representing the index or position of the block within the blockchain.
Timestamp: It is a DateTime property representing the timestamp or the time at which the block was created or added to the blockchain.
Data: It is a string property that holds the data or information associated with the block. The data can vary depending on the purpose of the blockchain.
PreviousHash: It is a string property that stores the hash value of the previous block in the blockchain. This property is used to maintain the integrity and chronological order of the blocks in the blockchain.
Hash: It is a string property that stores the hash value of the current block. The hash is calculated based on the combination of all the block’s properties (including Index, Timestamp, Data, and PreviousHash) and serves as a unique identifier for the block. It ensures the immutability and security of the block’s contents.
next step is to add a new class file called Blockchain.cs to represent the entire blockchain and provide the necessary methods for managing blocks.
public class Blockchain
{
private List<Block> chain;
public Blockchain()
{
chain = new List<Block>();
InitializeGenesisBlock();
}
private void InitializeGenesisBlock()
{
chain.Add(CreateGenesisBlock());
}
private Block CreateGenesisBlock()
{
return new Block
{
Index = 0,
Timestamp = DateTime.Now,
Data = "Genesis Block",
PreviousHash = string.Empty,
Hash = CalculateBlockHash(0, DateTime.Now, "Genesis Block", string.Empty)
};
}
private string CalculateBlockHash(int index, DateTime timestamp, string data, string previousHash)
{
string blockData = $"{index}-{timestamp}-{data}-{previousHash}";
byte[] bytes = Encoding.UTF8.GetBytes(blockData);
byte[] hashBytes;
using (SHA256 sha256 = SHA256.Create())
{
hashBytes = sha256.ComputeHash(bytes);
}
StringBuilder builder = new StringBuilder();
foreach (byte b in hashBytes)
{
builder.Append(b.ToString("x2"));
}
return builder.ToString();
}
}
Futhermore, the detailed explanation of the above code is as follows.
Block Class:
This class represents a block in the blockchain.
It contains properties such as Index, Timestamp, Data, PreviousHash, and Hash.
The properties store information about the block’s position, creation time, data, and hash values.
The Hash property is calculated based on the block’s properties and serves as a unique identifier.
Blockchain Class:
This class represents the blockchain itself and manages the chain of blocks.
It has a private member variable called chain, which is a list of Block objects representing the blocks in the chain.
Blockchain() Constructor:
This constructor initializes a new instance of the Blockchain class.
It creates an empty list for the chain and calls the InitializeGenesisBlock() method.
InitializeGenesisBlock() Method:
This method adds the genesis block (the first block in the blockchain) to the chain.
It calls the CreateGenesisBlock() method to create the genesis block and adds it to the chain list.
CreateGenesisBlock() Method:
This method creates and returns the genesis block.
It sets the properties of the genesis block, including the index, timestamp, data, previous hash, and calculates the hash value based on these properties using the CalculateBlockHash() method.
CalculateBlockHash() Method:
This method calculates and returns the hash value of a block.
It concatenates the index, timestamp, data, and previous hash into a single string.It converts the concatenated string to bytes and uses the SHA256 algorithm to compute the hash value.The hash bytes are then converted to a hexadecimal string representation and returned.
Overall the Blockchain class, it will automatically initialize with a genesis block.
we will add our class as a service in Program.cs services.AddSingleton(); next to test run the application to see the result in json Format.