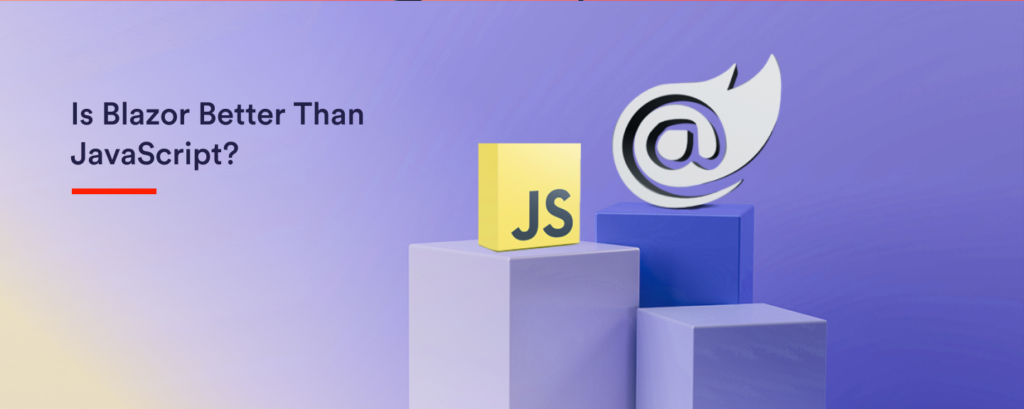
Are you tired of using JavaScript for building web applications? Have you ever wished that there was a better way to build web applications without having to deal with the complexities of JavaScript? Well, there is! Blazor is a new web development framework that allows developers to build interactive web applications using C# instead of JavaScript.
In this blog post, we will explore some of the reasons why Blazor is better than JavaScript for web development. We’ll discuss the advantages of using Blazor, including its simplified development process, increased performance, and code reusability etc.
What Does Blazor Offer?
Compatibility with various operating systems – The code which is written in Visual Studio improves overall Blazor app development and offers mixtures of operating systems like macOS, Linus, and Windows.
Exchanging .NET code and libraries -The Blazor based apps use the existing .NET libraries. All credit goes to the standardized format of .NET for building an official explicit .NET libraries and .NET code.
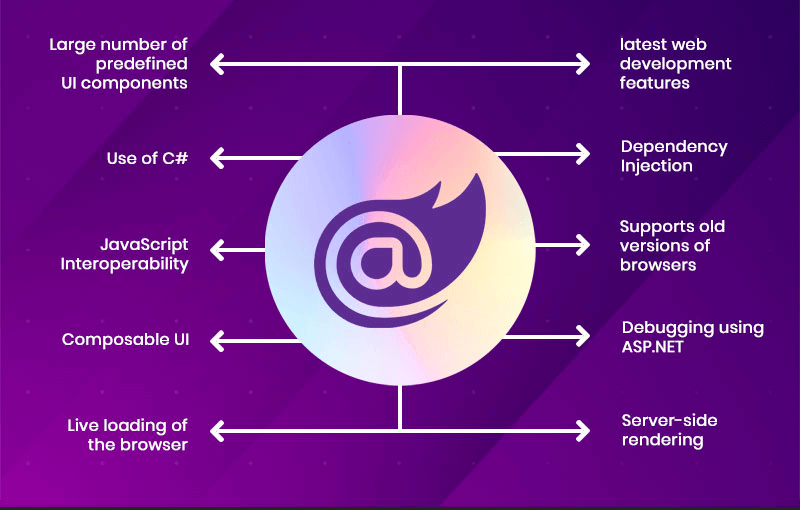
- Blazor shares the server-side code and client-side code: Blazor allows developers to reuse code between the front end and back end.
- Dependency injection: Dependency injection is a usable object that can act as a service in Blazor. Blazor uses dependency injection in its applications to achieve inversion of control. It allows providing objects with dependencies. In Blazor, dependency injection can be divided into classes: injector, client, and service. Blazor also features different injectors such as constructors, properties, and methods.
- Visual Studio Code: We can develop Blazor apps using visual studio code since both are Microsoft products. When you develop an app in the Blazor framework, VS Code will help you utilize its features easily.
- Interoperability with JavaScript: Blazor uses interop functionality in Blazor WebAssembly to handle DOM manipulations. Also, this JavaScript functionality can use browser API calls in Blazor WebAssembly. Therefore, Blazor apps can use .NET methods with JavaScript functions.
- Unrestricted access and open source: Blazor is connected to the open-source.NET platform, which has a strong and reliable network of almost 60,000 supporters from over 3,700 distinct companies.
While both Blazor and JavaScript can be used to build web applications, they have different strengths and use cases. Blazor is particularly useful for developers who are already familiar with C# and want to build web applications without having to learn JavaScript. JavaScript, on the other hand, is more widely used and has a larger community of developers and resources available. Ultimately, the choice between Blazor and JavaScript will depend on the specific requirements of your project and the skillset of your development team.
Blazor Vs React JS
Blazor and React are two different web development frameworks that can be used to build modern web applications.Blazor is a web framework developed by Microsoft that allows developers to build interactive web applications using C# instead of JavaScript, while React is a JavaScript library for building user interfaces.
One of the main differences between Blazor and React is the language used to build applications. Blazor uses C#, while React uses JavaScript. This means that developers who are already familiar with C# may find it easier to work with Blazor, while those who are more experienced with JavaScript may prefer to use React.
Another difference between Blazor and React is the way they handle user interface rendering. Blazor uses a server-side rendering model, where the HTML for the user interface is generated on the server and sent to the client, while React uses a client-side rendering model, where the HTML is generated on the client-side using JavaScript.
React | Blazor | |
Type | Front-End Library – focuses on the client UI and interactions of your website. | Full Framework – client side (WASM) and server side (ASP.NET) |
Developer | Microsoft | |
License | MIT | Apache |
Language | JavaScript/JSX/TypeScript | C# |
Performance | Light weight with great performance. | WASM client-side apps have a heavier first-time load. Server-side highly performant |
Learning Curve | Easy to Learn | Easy to Learn |
PWA (Progressive Web App) Support | Yes | Yes (Blazor WebAssembly) |
Routing | Not included | Yes |
Http Client | Not included | Yes |
Dependency Injection | Not included | Yes |
Requires an active connection per client | No | Yes |
Stores the component state server-side for each client | No | Yes (Blazor Server) |
Scoped styles for components | Yes | Yes |
Static Deployment | Yes | Yes (Blazor WebAssembly) |
Server-Side Rendering | Yes | Yes |
Optimized for SEO / Crawlers | Yes | Yes |
Bundle Size | 12KB gzipped, but only a client render framework, not full stack. | Minimal for Blazor Server. As low as 393kb, up to 780kb for .NET Framework in a Client-side WASM app. |
Tooling | CLI plus many 3rd party options. | CLI, Visual Studio and 3rd party options. |
Production Ready | React is production-ready today with years of battle-tested deployments from companies such as Uber, Drop Box, Twitter, Paypal, Netflix, Walmart, and more. | Yes, considering the major enhancements release in November 2021 with NET 6. |
Ultimately, the choice between Blazor and React will depend on the specific requirements of your project, the skillset of your development team, and the available resources and tools. Both frameworks have their strengths and use cases, and choosing the right one for your project will require careful consideration and evaluation.
Blazor Vs Angular JS
Blazor and Angular are two popular web development frameworks that can be used to build modern web applications. Blazor is a web framework developed by Microsoft that allows developers to build interactive web applications using C# instead of JavaScript, while Angular is a JavaScript framework for building dynamic, single-page web applications.
One of the main differences between Blazor and Angular is the language used to build applications. Blazor uses C#, while Angular uses TypeScript, which is a superset of JavaScript. This means that developers who are already familiar with C# may find it easier to work with Blazor, while those who are more experienced with JavaScript may prefer to use Angular.
Another difference between Blazor and Angular is the way they handle user interface rendering. Blazor uses a server-side rendering model, where the HTML for the user interface is generated on the server and sent to the client, while Angular uses a client-side rendering model, where the HTML is generated on the client-side using JavaScript.
Ultimately, the choice between Blazor and Angular will depend on the specific requirements of your project, the skillset of your development team, and the available resources and tools. Both frameworks have their strengths and use cases, and choosing the right one for your project will require careful consideration and evaluation.